Introduction
We all make mistakes, and, it’s my belief that we all make an honest attempt to correct that mistake. If only, life was running on Git. Git offers two ways to rollback a change made to a codebase – revert and rese. In this article we will discuss the two commands and the scenarios in which they are apt to be used.
Basic Terminology
Before we embark on discussing the differences between the commands, it is prudent to discuss some basic terminology that will be instrumental in understanding the nuances of operations offered by these commands.
HEAD: Represents the last commit and is a pointer to the current branch selected in git.
INDEX: Represents the staging area aka the contents of the proposed next commit.
Working Directory: Contains all the files available in HEAD and INDEX and the artifacts that may never go in any commit. E.g. Assembly files (.dll, .exe) which do not get committed due to .gitignore settings.
Revert command
Sometimes it happens that wrong work gets committed and is pushed into the remote repository, making it available to the rest of the team, or project contributors, as the case may be. In such circumstances, it becomes imperative, that we introduce a context under which a rollback of the unintentional changes take place.
Revert offers just such functionality. It creates an opposite commit of the commit that you want to roll back. Such a rollback commit retains a detailed history of all the commits that came before it, thus avoiding all the confusion.
Run the following commands in bash shell to simulate a revert:
$ mkdir Revert
$ cd Revert
$ git init
$ touch file.txt
$ printf “First commit”>file.txt
$ git add file.txt
$ git commit -m “First commit”
$ touch file2.txt
$ printf “Second commit”>file2.txt
$ git add file2.txt
$ git commit -m “Second commit”
$ printf “\n\nThird commit”>>file.txt
$ git add file.txt
$ git commit -m “Third commit”
$ dir
Note that there are two files file.txt and file2.txt in the INDEX and working directory.
$ git status
$ git log –oneline
Note there are three commits. Now let’s revert the second commit. Since the file2.txt was created as part of the second commit, it should get deleted from the working directory and INDEX
$ git revert SHA1 hash corresponding to the second commit.
$ dir
$ git log –oneline
$ git status
The result of following the aforementioned commands should look something like:
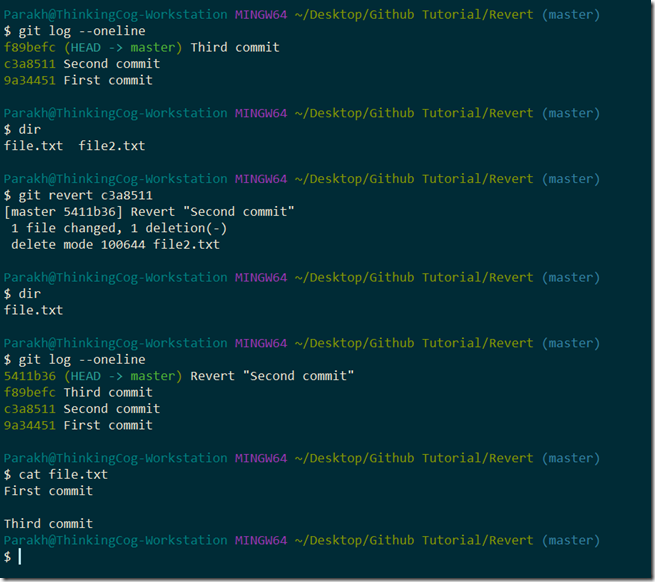
As you see, now we have a total of four commits in the history, with the fourth one clearly jotted down as a revert commit, erasing the work done as part of the second commit.
Reset command
Reset is used when you have the fault available locally on your system and has not been pushed out to the remote repository. Now this command offers a further three flavors and it will be here that we will be chiefly leveraging the terminology covered earlier.
Soft Reset: This option moves the branch (that HEAD points to) to the commit mentioned, reinstating the conditions as they were when the commit was made. No changes are introduced in the INDEX (staging area) and working directory. This essentially does the same this as “git commit –amend” and offers us a chance to change what we need to.
This means that you will get a chance to amend the commit message. Note that no changes are introduced into the file(s) made prior to the rolled back commit in soft mode.
Mixed Reset: This option moves the branch (that HEAD points to) and INDEX (staging area) to the commit mentioned, reinstating the conditions as they were when the commit was made. No changes are introduced in the working directory.
Hard Reset: This option moves the branch (that HEAD points to), INDEX (staging area) and the working area to the commit mentioned, reinstating the conditions as they were when the commit was made. This is destructive in nature and should be used with caution as work is not recoverable after the issuance of this command.
NOTE: In none of the cases HEAD is moved to the mentioned commit. HEAD always point to the branch. It is the branch that is made to point to the pointer corresponding to the commit being reset.
Run the following commands in bash shell to simulate all three flavors of reset:
$ mkdir Reset
$ cd Reset
$ git init
$ touch file.txt
$ printf “First commit” > file.txt
$ git add file.txt
$ git commit -m “First commit”
$ printf “\n\nSecond commit” >> file.txt
$ git add file.txt
$ git commit -m “Second commit”
$ printf “\n\nThird commit” >> file.txt
$ git add file.txt
$ git commit -m “Third commit”
$ git status
$ git log –oneline
$ git reset --soft head~1
Now observe the changes made by the soft reset command
$ status
$ git log --oneline
The following are the results of the soft reset:
1. The third commit has been rolled back as seen in the commit log,
2. No changes have been introduced in the INDEX i.e. the staging area. The git status helpfully suggests that file.txt is ready to be committed into the repository. The contents of the file.txt are the same as they existed prior to the rolled back commit,
3. No changes have been introduced in the working directory. All the contents are intact.
Let’s continue our example to mixed reset:
$ git add file.txt
$ git commit “Third commit”
$ git reset --mixed head~1
Now observe the changes made by the mixed reset command
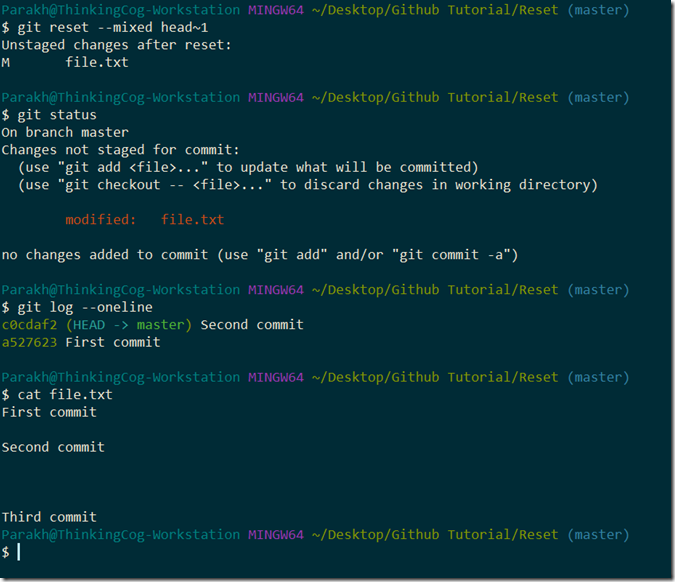
The following are the results of the mixed reset:
1. The third commit has been rolled back as seen in the commit log,
2. Changes have been introduced in the staging area, as suggested by the status. It helpfully suggests that file.txt needs to be added in order for it to be committed.
3. No changes have been introduced in the working directory.
Let’s continue our example to hard reset
$ git add file.txt
$ git commit “Third commit”
$ git reset --hard head~1
Now observe the changes made by the hard reset command
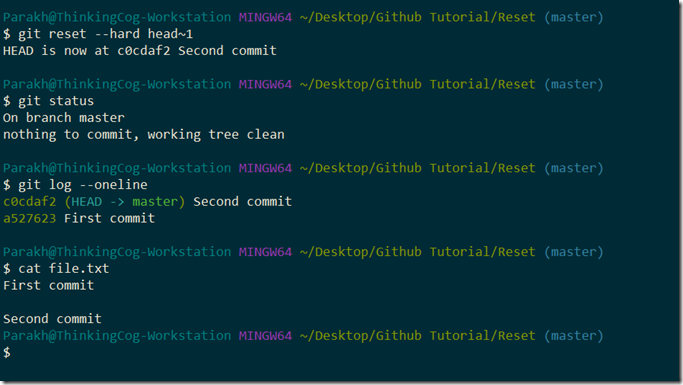
The following are the results of the hard reset:
1. The third commit has been rolled back as seen in the commit log,
2. INDEX i.e. staging area has been put into the state as it existed after the second commit and prior to the third commit. The data corresponding to the third commit has been lost from the file.txt.
3. Working directory has been put into the state as it existed prior to the third commit. Any contents introduced between the second and the third commit would have been lost as part of the hard reset of the third commit.
Conclusion
Now that we have seen the difference between revert and reset and when the two should be used. We also saw the three modes made available by revert - soft, mixed and hard reset and how they affect the state of the working directory, INDEX and the content. Hard reset should be used with caution since the content is not recoverable after the issuance of the command.
References
1. https://git-scm.com/book/en/v2
2. https://www.atlassian.com/git/tutorials/resetting-checking-out-and-reverting